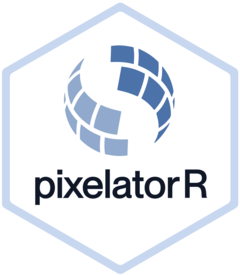
Get and set CellGraph object data
CellGraphData.Rd
Get and set CellGraph object data
Arguments
- object
A
CellGraph
object- slot
Information to pull from object (cellgraph, meta_data, layout)
- value
A new variable to place in
slot
Examples
library(pixelatorR)
library(dplyr)
#>
#> Attaching package: ‘dplyr’
#> The following objects are masked from ‘package:stats’:
#>
#> filter, lag
#> The following objects are masked from ‘package:base’:
#>
#> intersect, setdiff, setequal, union
library(tidygraph)
#>
#> Attaching package: ‘tidygraph’
#> The following object is masked from ‘package:stats’:
#>
#> filter
edge_list <-
ReadMPX_item(
minimal_mpx_pxl_file(),
items = "edgelist"
)
#> ℹ Loading item(s) from: /private/var/folders/gw/bdcqhnvs0m9gs_mq8n51jtbc0000gn/T/Rtmp9yI9aG/temp_libpath1491327224194/pixelatorR/extdata/five_cells/five_cells.pxl
#> → Loading edgelist data
#> ✔ Returning a 'tbl_df' object
bipart_graph <-
edge_list %>%
select(upia, upib, marker) %>%
distinct() %>%
as_tbl_graph(directed = FALSE) %>%
mutate(node_type = case_when(name %in% edge_list$upia ~ "A", TRUE ~ "B"))
attr(bipart_graph, "type") <- "bipartite"
cg <- CreateCellGraphObject(cellgraph = bipart_graph)
# Get slot data
CellGraphData(cg, slot = "cellgraph")
#> # A tbl_graph: 16800 nodes and 68255 edges
#> #
#> # An undirected multigraph with 5 components
#> #
#> # Node Data: 16,800 × 2 (active)
#> name node_type
#> <chr> <chr>
#> 1 GGTATATATTTTAAGTAGTTTAGTA A
#> 2 ATTATGTTGTTGTATGTTTATTATT A
#> 3 TCCGTGGTTTGATACTCGGGAATTT A
#> 4 AGTGTAAGAGGTTGTTTCTTAGAAA A
#> 5 GAGCAGACAATGGCGCTTAGCTAAA A
#> 6 CAATTTTGACCTAGTTGTGGCCAAG A
#> 7 ATGTCAGAGTGAATAGTTGTGATTG A
#> 8 ATAAAGTGACCAGTTGAATGGGCCC A
#> 9 TGCCTCTTGAGCTGTTGATCGCCTG A
#> 10 ATTAGATGTAGAGCGCGAAAATTGG A
#> # ℹ 16,790 more rows
#> #
#> # Edge Data: 68,255 × 3
#> from to marker
#> <int> <int> <chr>
#> 1 1 11034 CD27
#> 2 2 11035 CD27
#> 3 3 11036 CD27
#> # ℹ 68,252 more rows
# Set slot data
CellGraphData(cg, slot = "cellgraph") <- CellGraphData(cg, slot = "cellgraph")