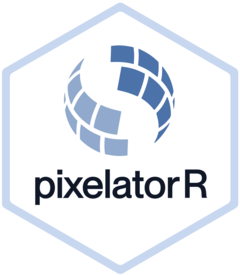
Type check helpers
type_check_helpers.Rd
Utility functions for type checking in pixelatorR
. These functions
throw informative error messages if the checks fail.
Usage
assert_single_value(
x,
type = c("string", "numeric", "integer", "bool"),
allow_null = FALSE,
arg = caller_arg(x),
call = caller_env()
)
assert_vector(
x,
type = c("character", "numeric", "integer", "logical"),
n = 2,
allow_null = FALSE,
arg = caller_arg(x),
call = caller_env()
)
assert_class(
x,
classes,
allow_null = FALSE,
arg = caller_arg(x),
call = caller_env()
)
assert_mpx_assay(
x,
allow_null = FALSE,
arg = caller_arg(x),
call = caller_env()
)
assert_pna_assay(
x,
allow_null = FALSE,
arg = caller_arg(x),
call = caller_env()
)
assert_pixel_assay(
x,
allow_null = FALSE,
arg = caller_arg(x),
call = caller_env()
)
assert_within_limits(x, limits, arg = caller_arg(x), call = caller_env())
assert_function(
x,
allow_null = FALSE,
arg = caller_arg(x),
call = caller_env()
)
assert_file_exists(x, allow_null = FALSE, call = caller_env())
assert_file_ext(x, ext, allow_null = FALSE, call = caller_env())
assert_x_in_y(
x,
y,
allow_null = FALSE,
arg_x = caller_arg(x),
arg_y = caller_arg(y),
call = caller_env()
)
assert_single_values_are_different(
x,
y,
allow_null = FALSE,
arg_x = caller_arg(x),
arg_y = caller_arg(y),
call = caller_env()
)
assert_singles_match(
x,
y,
arg_x = caller_arg(x),
arg_y = caller_arg(y),
call = caller_env()
)
assert_length(
x,
n = 1,
allow_null = FALSE,
arg_x = caller_arg(x),
call = caller_env()
)
assert_max_length(
x,
n = 1,
allow_null = FALSE,
arg_x = caller_arg(x),
call = caller_env()
)
assert_vectors_x_y_length_equal(
x,
y,
arg_x = caller_arg(x),
arg_y = caller_arg(y),
call = caller_env()
)
assert_unique(x, arg = caller_arg(x), call = caller_env())
assert_col_class(
x,
data,
classes,
allow_null = FALSE,
arg_data = caller_arg(data),
call = caller_env()
)
assert_col_in_data(
x,
data,
allow_null = FALSE,
arg_data = caller_arg(data),
call = caller_env()
)
assert_non_empty_object(
x,
classes,
allow_null = FALSE,
arg = caller_arg(x),
call = caller_env()
)
assert_is_one_of(
x,
choices,
allow_null = FALSE,
arg = caller_arg(x),
call = caller_env()
)
assert_different(
x,
y,
allow_null = FALSE,
arg_x = caller_arg(x),
arg_y = caller_arg(y),
call = caller_env()
)
assert_vectors_match(
x,
y,
allow_null = FALSE,
arg_x = caller_arg(x),
arg_y = caller_arg(y),
call = caller_env()
)
assert_valid_color(
x,
n = 1,
allow_null = FALSE,
arg = caller_arg(x),
call = caller_env()
)
Arguments
- x, y
An object to check
- type
A character string specifying the type or vector class to check against.
- allow_null
Either
TRUE
orFALSE
. IfTRUE
,x
can beNULL
and the check passes.- arg, arg_x, arg_y, arg_data
The name of an argument to check. Used for error messages.
- call
An environment, typically the environment in which the function was called
- n
An integer
- classes
A character vector of classes to check against
- limits
A numeric vector of length 2 specifying a lower and upper limit
- ext
A character string specifying a file extension
- data
A data-frame like object
- choices
A vector of allowed values
Details
assert_single_value
checks ifx
is a single value of a specifiedtype
.assert_vector
checks ifx
is a vector of a specifiedtype
and with at leastn
elements.assert_class
checks ifx
is of a specific class.assert_mpx_assay
checks ifx
is aCellGraphAssay
orCellGraphAssay5
.assert_pna_assay
checks ifx
is aPNAAssay
orPNAAssay5
.assert_pixel_assay
checks ifx
is aCellGraphAssay
,CellGraphAssay5
,PNAAssay
orPNAAssay5
.assert_x_in_y
checks if all elements ofx
are iny
.assert_single_values_are_different
checks ifx
andy
are not different strings.assert_col_class
checks if columnx
indata
is of a specific class.assert_col_in_data
checks if columnx
is present indata
.assert_vectors_match
checks if vectorsx
andy
are identical.assert_length
checks if vectorx
has a specific length.assert_within_limits
checks if values inx
are withinlimits
.assert_function
checksx
is a function.assert_file_exists
checks is filex
exists.assert_file_ext
checks is filex
has file extensionext
.assert_unique
checks if values inx
are unique.