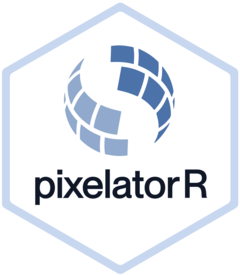
Differential analysis (abundance)
RunDAA.Rd
Runs differential analysis on abundance values generated with the
Pixelator
data processing pipeline. The function is a wrapper around
the FindMarkers
function from Seurat
but provides a similar
interface as RunDPA
and RunDCA
from pixelatorR
.
Currently, RunDAA
only has a method for Seurat
objects.
Usage
RunDAA(object, ...)
# S3 method for class 'Seurat'
RunDAA(
object,
contrast_column,
reference,
targets = NULL,
assay = NULL,
group_vars = NULL,
mean_fxn = Matrix::rowMeans,
fc_name = "difference",
p_adjust_method = c("bonferroni", "holm", "hochberg", "hommel", "BH", "BY", "fdr"),
verbose = TRUE,
...
)
Arguments
- object
A Seurat object containing abundance data, such as counts or normalized counts
- ...
Parameters passed to
FindMarkers
- contrast_column
The name of the meta data column where the group labels are stored. This column must include
targets
andreference
.- reference
The name of the reference group
- targets
The names of the target groups. These groups will be compared to the reference group. If the value is set to
NULL
(default), all groups available incontrast_column
exceptreference
will be compared to thereference
group.- assay
Name of assay to use
- group_vars
An optional character vector with column names to group the tests by.
- mean_fxn
Function to use for fold change or average difference calculation in
FindMarkers
. See documentation for themean.fxn
parameter inFindMarkers
for more information.- fc_name
Name of the fold change, average difference, or custom function column in the output tibble. See documentation for the
fc.name
parameter inFindMarkers
for more information.- p_adjust_method
One of "bonferroni", "holm", "hochberg", "hommel", "BH", "BY" or "fdr". (see
?p.adjust
for details). The p values are adjusted across all tests and the replaces thep_val_adj
column generated byFindMarkers
.- verbose
Print messages
Details
The input object should contain a contrast_column
(character vector or factor)
that includes information about the groups to compare. A typical example is a column
with sample labels, for instance: "control", "stimulated1", "stimulated2". If the input
object is a Seurat
object, the contrast_column
should be available in
the meta.data
slot. For those familiar with FindMarkers
from Seurat,
contrast_column
is equivalent to the group.by
parameter.
The targets
parameter specifies a character vector with the names of the groups
to compare reference
. targets
can be a single group name or a vector of
group names while reference
can only refer to a single group. Both targets
and reference
should be present in the contrast_column
. These parameters
are similar to the ident.1
and ident.2
parameters in FindMarkers
.
Additional groups
The test is always computed between targets
and reference
, but it is possible
to add additional grouping variables with group_vars
. If group_vars
is used,
each comparison is split into groups defined by the group_vars
. For instance, if we
have annotated cells into cell type populations and saved these annotations in a meta.data
column called "cell_type", we can pass "cell_type" to group_vars="cell_type"
to split
tests across each cell type.
Types of comparisons
Consider a scenario where we have a Seurat object (seurat_object
) with PNA data.
seurat_object
contains a meta.data
column called "sampleID" that holds
information about what samples the PNA components originated from. This column could have
three sample IDs: "control", "stimulated1" and "stimulated2". In addition, we have a column
called "cell_type" that holds information about the cell type identity of each PNA component.
If we want to compare the "stimulated1" group to the "control" group:
daa_markers <- RunDAA(object = seurat_object, contrast_column = "sampleID", reference = "control", targets = "stimulated1")
If we want to compare the "stimulated1" and "stimulated2" groups to the "control" group:
If we want to compare the "stimulated1" and "stimulated2" groups to the "control" group, and split the tests by cell type:
See also
Other DA-methods:
DifferentialProximityAnalysis()
,
RunDCA()
,
RunDPA()
Examples
library(pixelatorR)
library(dplyr)
library(SeuratObject)
pxl_file <- minimal_mpx_pxl_file()
# Seurat objects
se <- ReadMPX_Seurat(pxl_file)
#> ✔ Created a 'Seurat' object with 5 cells and 80 targeted surface proteins
se <- merge(se, rep(list(se), 9), add.cell.ids = LETTERS[1:10])
se$sample <- c("T", "C", "C", "C", "C") %>% rep(times = 10)
se <- Seurat::NormalizeData(se %>% JoinLayers(), normalization.method = "CLR", margin = 2)
#> Normalizing layer: counts
#> Normalizing across cells
# Run DAA
daa_markers <- RunDAA(se,
contrast_column = "sample",
targets = "T", reference = "C"
)
#> For a (much!) faster implementation of the Wilcoxon Rank Sum Test,
#> (default method for FindMarkers) please install the presto package
#> --------------------------------------------
#> install.packages('devtools')
#> devtools::install_github('immunogenomics/presto')
#> --------------------------------------------
#> After installation of presto, Seurat will automatically use the more
#> efficient implementation (no further action necessary).
#> This message will be shown once per session
daa_markers
#> # A tibble: 80 × 8
#> marker p p_adj difference pct_1 pct_2 target reference
#> <chr> <dbl> <dbl> <dbl> <dbl> <dbl> <chr> <chr>
#> 1 CD137 0.000000407 0.0000326 0.0568 1 0.5 T C
#> 2 CD62P 0.000000792 0.0000633 -0.130 0 1 T C
#> 3 CD11b 0.000000792 0.0000633 0.0400 1 0.75 T C
#> 4 CD152 0.000000792 0.0000633 0.218 1 0.75 T C
#> 5 CD163 0.000000792 0.0000633 0.0641 1 0.75 T C
#> 6 CD200 0.000000792 0.0000633 0.236 1 0.75 T C
#> 7 CD154 0.000000792 0.0000633 0.111 1 0.75 T C
#> 8 CD328 0.000000792 0.0000633 0.161 1 0.75 T C
#> 9 CD158 0.000000792 0.0000633 0.215 1 0.75 T C
#> 10 CD279 0.000000792 0.0000633 0.843 1 1 T C
#> # ℹ 70 more rows